In this tutorial, we are going to guide you on your first interaction with React JS.
Nowadays, in the world of web development we keep on hearing the world "Hey, I'm using react what's yours?" or something like "React vs Vue.js vs Angular, which one is the best?".
Well, whatever you choose, it will have the same output it will only depend on how you use it. So don't get confused about which one to use, Just learn it.
What is React?
- It's an open-source javascript library to build interactive interfaces and dynamic contents without refreshing the page.
- It allows us to create a reusable UI components that you can use anywhere in your application.
- React main purpose is to be fast, scalable and simple.
Why React?
- We can skip this part. It's a never-ending "WHY" question.
- Let's just proceed in our tutorial.
Prerequisites of React
- You should know at least the basic fundamentals of HTML, CSS, and Javascript.
- You should also be aware of ES6.
- Willingness to learn.
Okay, so how do we use it?
To use react we need to make sure that your system has the basic requirements.
- NodeJS
- NPM
Incase you have already installed it, you may skip this part.
Option 1: Static Html File ( Traditional )
If ever you just want to try react in your static html you can do that too. Please check our codes below.
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8" />
<title>Welcome to Static HTML React! - Let's Code Pare</title>
<!-- Bootstrap CSS -->
<link rel="stylesheet" href="https://stackpath.bootstrapcdn.com/bootstrap/4.3.1/css/bootstrap.min.css" integrity="sha384-ggOyR0iXCbMQv3Xipma34MD+dH/1fQ784/j6cY/iJTQUOhcWr7x9JvoRxT2MZw1T" crossorigin="anonymous">
</head>
<body>
<main role="main">
<section class="jumbotron text-center">
<div class="container">
<!-- Normal Html file -->
<h1 class="jumbotron-heading">
<a href="https://letscodepare.com/blog/category/react" title="Explore React Tutorials"> Explore React Tutorials</a>
</h1>
<p class="lead text-muted">
Education matters, never stop learning new skills
<br/> <small>Learn react and build your own app</small>
</p>
<!-- REACT START -->
<!-- it will be our root container for our main react code -->
<div id="lcp-root"></div>
<!-- REACT END -->
</div>
</section>
</main>
<script type="text/babel">
// React code will go here
class App extends React.Component {
render() {
return <h1>Welcome to React World!</h1>
}
}
ReactDOM.render(<App />, document.getElementById('lcp-root'))
</script>
<!-- Include Bootstrap -->
<!-- Not connected to react js -->
<script src="https://code.jquery.com/jquery-3.3.1.slim.min.js" integrity="sha384-q8i/X+965DzO0rT7abK41JStQIAqVgRVzpbzo5smXKp4YfRvH+8abtTE1Pi6jizo" crossorigin="anonymous"></script>
<script src="https://cdnjs.cloudflare.com/ajax/libs/popper.js/1.14.7/umd/popper.min.js" integrity="sha384-UO2eT0CpHqdSJQ6hJty5KVphtPhzWj9WO1clHTMGa3JDZwrnQq4sF86dIHNDz0W1" crossorigin="anonymous"></script>
<script src="https://stackpath.bootstrapcdn.com/bootstrap/4.3.1/js/bootstrap.min.js" integrity="sha384-JjSmVgyd0p3pXB1rRibZUAYoIIy6OrQ6VrjIEaFf/nJGzIxFDsf4x0xIM+B07jRM" crossorigin="anonymous"></script>
<!-- React JS Dependencies -->
<script src="https://unpkg.com/react@^16/umd/react.production.min.js"></script>
<script src="https://unpkg.com/react-dom@^16/umd/react-dom.production.min.js"></script>
<script src="https://unpkg.com/babel-standalone@^6/babel.js"></script>
</body>
</html>
I'm loading the CDN file for the latest versions required to run react in your browser.
- React - the React top level API
- React DOM - control the contents of the container inside dom
- Babel - a JavaScript compiler
Once you run your this html file, the output will be like this
Option 2: Installation Procedure (Recommended)
- Go to nodejs.org website and download the installer based in your system.
- Once you downloaded it, install it and follow the installation process.
- If installation is successful, open your terminal or command prompt and let's verify the node version.
node -v
# it should display the current version of node you installed.
#ex. v10.21.0
npm -v
# check npm version, this command will help you install different plugins.
#ex. 6.14.4
Congratulations! You’ve just installed a powerful tool to help you build an amazing web project.
So now, can we make a working application?
Open up your favorite terminal and type this code, and follow the wizard screen process.
# Intialize npm package
npm init
It will look like this:
package name: (codes) letscodepare
version: (1.0.0)
description:
entry point: (index.js)
test command: none
git repository:
keywords:
author:
license: (ISC)
It will create a package.json which contain all our npm packages.
Still there?
Let's install our react packages. Basically we only need to install three (3) packages to run our first ever react application.
- react - react is a javaScript library for building user interfaces.
- react-dom - react package for working with the DOM.
- react-scripts - create single page react without installing any babel or webpack configuration.
Type this in your terminal, it will install all the dependencies needed for us to start.
npm install --save react react-dom react-scripts
Once everything is installed, let's create a few folders and files to setup our react application.
Create folder and named it:
- /public
- /src
Inside /public folder, create a file and named it index.html and add this short html codes.
Almost same as our static html file, but no libraries or any javascript dependency will be loaded in this file.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Beginners Guide Using React - Let's Code Pare</title>
</head>
<body>
<noscript>You need to enable JavaScript to run this app.</noscript>
<div id="lcp-root"></div>
</body>
</html>
Go ahead and create new file inside /src folder
- /src/app.js
- /src/index.js
Now in /index.js, we're importing react and react-dom modules.
import React from 'react';
import ReactDOM from 'react-dom';
Let's create our /app.js code,
import React from 'react';
function App() {
return (
<div className="App">
Beginners guide using react by Let's Code Pare
</div>
);
}
export default App;
Note: If you notice, inside our
<div>
element we use "className" attribute instead of "class". When adding html attribute you should follow the camelCase naming convention using react. Ex. you have tab-index attribute, instead of writing it like this<div tab-index="0"></div>
you will write it like this<div tabIndex="0"></div>
.
Almost There?
Yes, we're almost there just a few more line of codes.
Now, we are going to connect our /app.js and /index.js Open up your /src/index.js file and import /app.js inside.
import React from 'react';
import ReactDOM from 'react-dom';
// import app.js like this.
import App from './app';
Finally, we'll render the App to the root document using react-dom.
ReactDOM.render(
<App />,
document.getElementById('lcp-root') // div id name inside of our index.html
);
The complete /index.js file code
import React from 'react';
import ReactDOM from 'react-dom';
import App from './app';
ReactDOM.render(
<App />,
document.getElementById('lcp-root')
);
The moment of truth
To finally run your react script, we're going to setup our react-script to open a port and start a local server.
Open your /package.json file, inside scripts
tag input this code.
"scripts": {
"start": "react-scripts start"
},
Then, open up again your terminal and at the root of your project run this command
npm start
It will start a development server by default it will run in your port 3000
Open your browser and point it to
http://localhost:3000
Voila!!!
You setup your first ever react application. I know, by it looks it's not good so let's try to apply boostrap like what we did in our static html file in option 1.
Add this boostrap css inside the <head></head>
tag.
<!-- Bootstrap CSS -->
<link rel="stylesheet" href="https://stackpath.bootstrapcdn.com/bootstrap/4.3.1/css/bootstrap.min.css" integrity="sha384-ggOyR0iXCbMQv3Xipma34MD+dH/1fQ784/j6cY/iJTQUOhcWr7x9JvoRxT2MZw1T" crossorigin="anonymous">
And wrap our <div id="lcp-root"></div>
inside a main container with bootstrap classes.
<main role="main">
<section class="jumbotron text-center">
<div class="container">
<!-- Normal Html file -->
<h1 class="jumbotron-heading">
<a href="https://letscodepare.com/blog/category/react" title="Explore React Tutorials"> Explore React Tutorials</a>
</h1>
<p class="lead text-muted">
Education matters, never stop learning new skills
<br/> <small>Learn react and build your own app</small>
</p>
<!-- REACT START -->
<!-- it will be our root container for our main react code -->
<div id="lcp-root"></div>
<!-- REACT END -->
</div>
</section>
</main>
Stay tune for our next tutorial.
Github Link
Voila!!!
I hope you enjoy our tutorial, Let me know incase you encounter any error I would love to answer that. Don't forget to subscribe to my Youtube Channel at Let's Code Pare - Youtube Channel
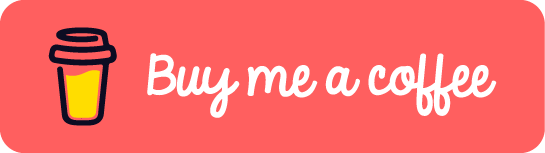